C Sharp ARTICLE
History of C Sharp
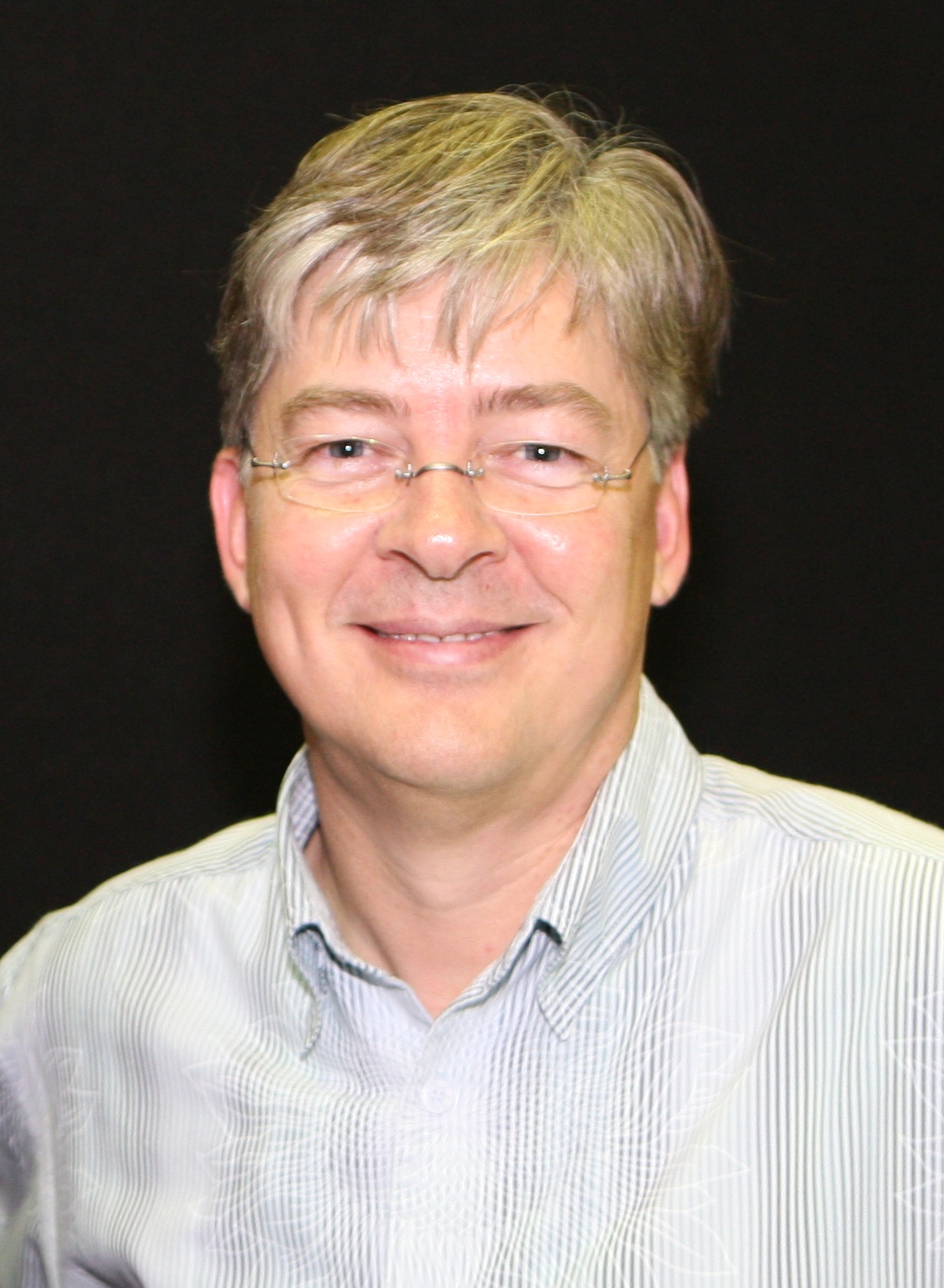
C Sharp programming language was designed by Anders Hejlsberg from Microsoft in 2000 and was later approved as an international standard by Ecma (ECMA-334) in 2002 and ISO/IEC (ISO/IEC 23270) in 2003. Microsoft introduced C Sharp along with .NET Framework and Visual Studio, both of which were closed-source.
C Sharp Introduction
What is C Sharp?
- C Sharp (pronounced "See Sharp") is a modern, object-oriented, and type-safe programming language.
- C Sharp enables developers to build many types of secure and robust applications that run in .NET.
- C Sharp has its roots in the C family of languages and will be immediately familiar to C, C++, Java, and JavaScript programmers.
- C# has added features to support new workloads and emerging software design practices.
C Sharp Syntax
- we can use classes from the System namespace.
- A blank line. C# ignores white space.
- namespace is used to organize your code, and it is a container for classes and other namespaces.
- The curly braces {} marks the beginning and the end of a block of code.
- class is a container for data and methods, which brings functionality to your program. Every line of code that runs in C# must be inside a class.
- Another thing that always appear in a C# program, is the Main method. Any code inside its curly brackets {} will be executed.
- Console is a class of the System namespace, which has a WriteLine() method that is used to output/print text.
- Note : Every C Sharp statement ends with a semicolon ;
Example:
using System;
namespace HelloWorld
{
class Program
{
static void Main ( string[] args )
{
Console.WriteLine ( "Welcome to BUIE-Learning" ) ;
}
}
}
Result: Welcome to BUIE-Learning
C Sharp Variables
- In C Sharp, there are different types of variables, for example:
- int: stores integers (whole numbers), without decimals, such as 123 or -123
- char: stores single characters, such as 'a' or 'B'.
- string: stores text, such as "BUIE Learning".
- double: stores floating point numbers, with decimals.
- bool: stores values with two states: true or false
Example:
int num = 5;
double DoubleNum = 5.99D;
char C = 'D';
bool Bool = true;
string myText = "Welcome";
Example of C sharp programming
Add Two Numbers
static void Main(string[] args )
{
int num1, num2, sum;
Console.WriteLine (" How to Calculate the sum of two numbers:");
Console.WriteLine(" Input number 1: " );
num1 = Convert.ToInt32( Console.ReadLine());
Console.Write(" Input number 2: " );
num2 = Convert.ToInt32(Console.ReadLine() );
sum = num1 + num2;
Console.Write(" {0} + {1} = {2}",num1,num2,sum);
Console.ReadKey();
}
Result:
How to Calculate the sum of two numbers:
Input number 1: 5
Input number 2: 10
5 + 10 = 15