KOTLIN ARTICLE
History of Kotlin
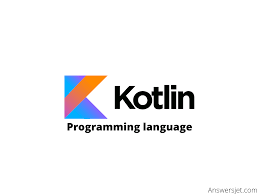
Kotlin was developed by JetBrains in 2010. They initially released it under the name Project Kotlin in July 2011. They needed a language that was concise, elegant, expressive, and also interoperable with Java, as most of their products were developed in Java, including the Intellij Idea.
Kotlin Introduction
What is Kotlin?
- Kotlin is an open-source statically typed programming language that targets the JVM, Android, JavaScript and Native. It’s developed by JetBrains
- Kotlin is a cross-platform, statically typed, general-purpose programming language with type inference.
kotlin Syntax
Program entry point
- An entry point of a Kotlin application is the main function.
fun main() {
println ("Hello world!")
}
Print to the standard output
- print prints its argument to the standard output.
print ("Hello ")
print ("world!")
Variables
- Read-only local variables are defined using the keyword val. They can be assigned a value only once.
val a: Int = 1 // immediate assignment
val b = 2 // `Int` type is inferred
val c: Int // Type required when no initializer is provided
c = 3 // deferred assignment
- Variables that can be reassigned use the var keyword.
var x = 5 // `Int` type is inferred
x += 1
- You can declare variables at the top level.
val PI = 3.14
var x = 0
fun incrementX() {
x += 1
}