Nodejs ARTICLE
History of Nodejs
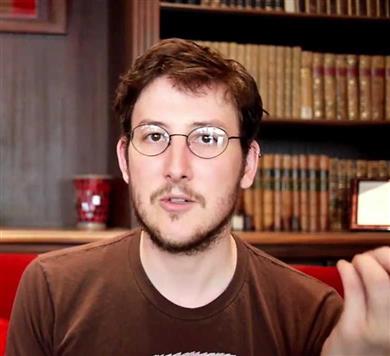
The open source project Node. js was invented by Joyent software engineer Ryan Dahl three years ago next month. It essentially allows JavaScript to be used outside of a browser.Node leverages Google's V8 JavaScript virtual machine to interpret JavaScript, and it uses an event-driven non-blocking I/O model that cloud services vendor Joyent -- a principal Node advocate -- says makes it ideal for data-intensive and real-time applications running across distributed devices. It is also championed by companies such as Microsoft and Mozilla.
Nodejs Introduction
What is Nodejs?
- Node.js is an open source, cross-platform runtime environment for developing server-side and networking applications. Node.js applications are written in JavaScript, and can be run within the Node.js runtime on OS X, Microsoft Windows, and Linux.
Example
Node.js = Runtime Environment + JavaScript Library
Features of Node.js
- Single Threaded but Highly Scalable-Node.js uses a single threaded model with event looping. Event mechanism helps the server to respond in a non-blocking way and makes the server highly scalable as opposed to traditional servers which create limited threads to handle requests.
- Very Fast -Being built on Google Chrome's V8 JavaScript Engine, Node.js library is very fast in code execution.
- No Buffering -Node.js applications never buffer any data. These applications simply output the data in chunks.
Nodejs Example
- Nodejs tool makes it easy to learn Node.js
var http = require('http');
http.createServer(function (req, res) {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello World!');
}).listen(8080);
Hello World!
Object Literal
- Object literal syntax is same as browser's JavaScript.
var obj = {
authorName: 'Ryan Dahl',
language: 'Node.js'
}
Functions
- Functions are first class citizens in Node's JavaScript, similar to the browser's JavaScript. A function can have attributes and properties also. It can be treated like a class in JavaScript.
Example
function Display(x) {
console.log(x);
}
Display (100);