Reactjs ARTICLE
History of Reactjs
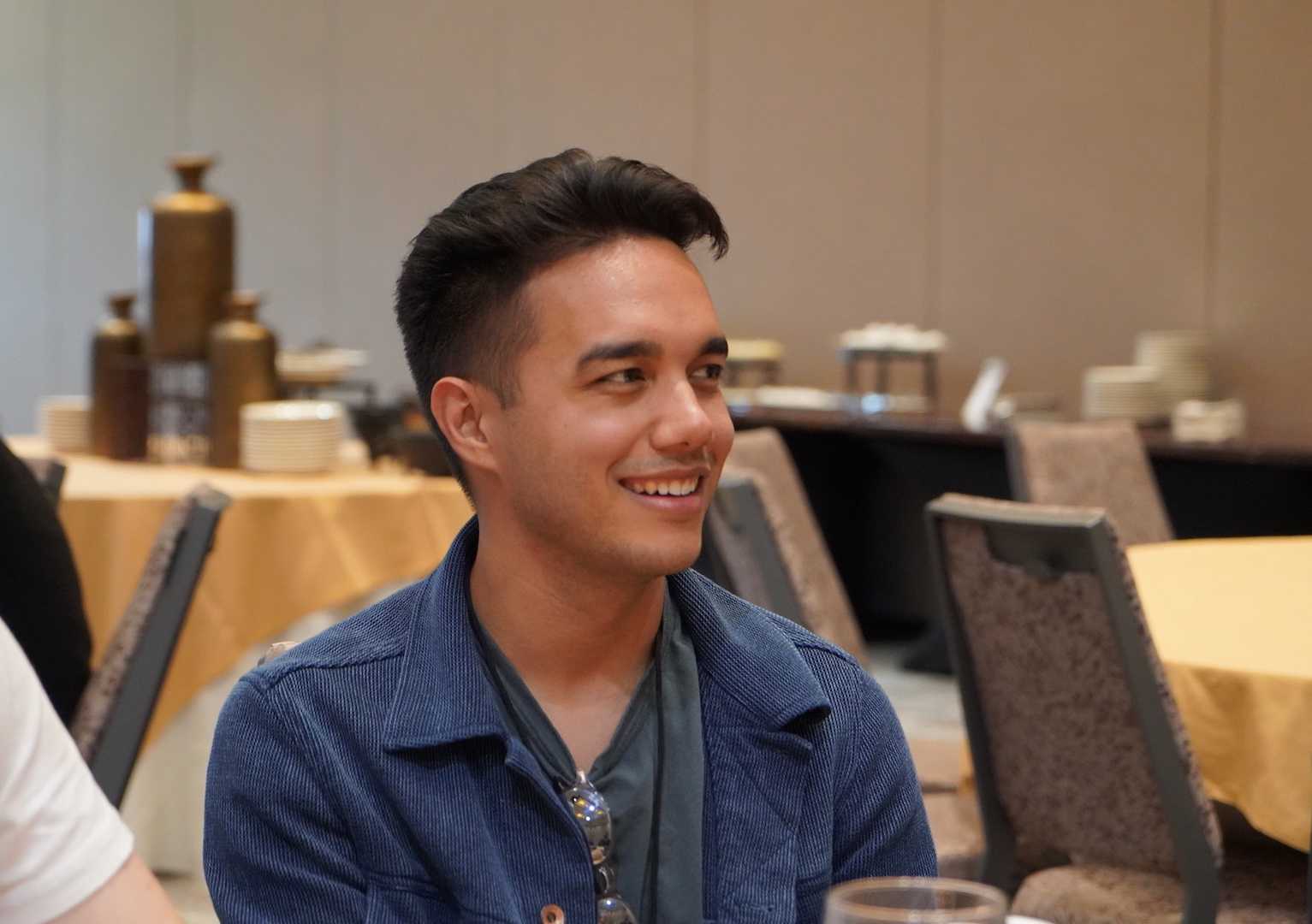
React is a free and open-source front-end JavaScript library for building user interfaces based on UI components. It is maintained by Meta and a community of individual developers and companies. It is developed by Jordan Walke. ReactJS is a declarative, efficient, and flexible JavaScript library for building reusable UI components.
ReactJS Introduction
What is ReactJS?
- React, sometimes referred to as a frontend JavaScript framework, is a JavaScript library created by Facebook. React is a tool for building UI components.
React Features
- Components − React is all about components. You need to think of everything as a component. This will help you maintain the code when working on larger scale projects.
- JSX − JSX is JavaScript syntax extension. It isn't necessary to use JSX in React development, but it is recommended.
- License − React is licensed under the Facebook Inc. Documentation is licensed under CC BY 4.0.
Advantages of ReactJS
- Uses virtual DOM which is a JavaScript object. This will improve apps performance, since JavaScript virtual DOM is faster than the regular DOM.
- Can be used on client and server side as well as with other frameworks.
- Component and data patterns improve readability, which helps to maintain larger apps.
Embedding Expressions in JSX
- In the example below, we declare a variable called name and then use it inside JSX by wrapping it in curly braces:
const name = 'Josh Perez';
const element = <h1>Hello, {name} </h1>;
- In the example below, we embed the result of calling a JavaScript function, formatName(user), into an <h1> element.
function formatName(user){
return user.firstName + ' ' + user.lastName;
}
const user = {
firstName: 'Harper',
lastName: 'Perez'
};
const element = (
<h1>
Hello, {formatName(user)}!
</h1>
);