Swift ARTICLE
History of Swift
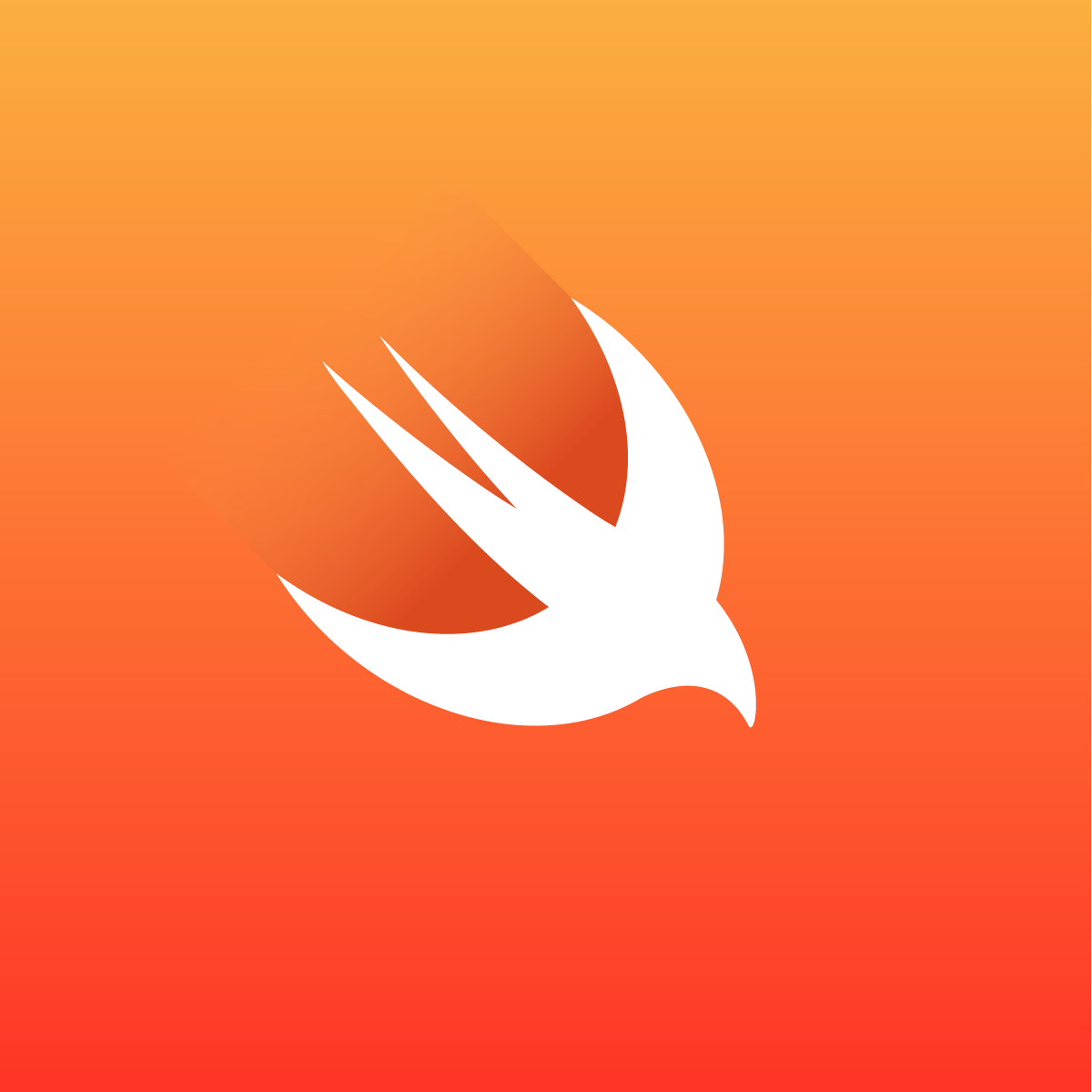
Swift is a general-purpose, multi-paradigm, compiled programming language developed by Apple Inc. and the open-source community. First released in 2014, Swift was developed as a replacement for Apple's earlier programming language Objective-C, as Objective-C had been largely unchanged since the early 1980s and lacked modern language features. Swift works with Apple's Cocoa and Cocoa Touch frameworks, and a key aspect of Swift's design was the ability to interoperate with the huge body of existing Objective-C code developed for Apple products over the previous decades.
Swift Introduction
What is Swift?
- ISO 9362 defines a standard format of Business Identifier Codes approved by the International Organization for Standardization.
- It is a unique identification code for both financial and non-financial institutions
- The acronym SWIFT stands for the Society for Worldwide Interbank Financial Telecommunication.
Swift Syntax
- Let's start once again with the following Hello, World! program created for OS X playground, which includes import Cocoa as shown below −
/* My first program in Swift 4 */
var myString = "Hello, World!"
print ( myString )
- If you create the same program for iOS playground, then it will include import UIKit and the program will look as follows −
import UIKit
var myString = "Hello, World!"
print ( myString )
Printing in Swift
- To print anything in swift we have ‘ print ‘ keyword.
- Print has three different properties.
- Items – Items to be printed
- Separator – separator between items
- Terminator – the value with which line should end, let’s see a example and syntax of same.
print ( "Items to print", separator: "Value " , terminator: "Value" )
print ( "Value one")
print ( "Value one","Value two", separator: " Next Value" , terminator: " End" )
Swift Variables
- Swift 4 supports the some variables −
- Float − This is used to represent a 32-bit floating-point number. It is used to hold numbers with smaller decimal points.Example-2.444 & -4.667
- Int or UInt − This is used for whole numbers. More specifically, you can use Int32, Int64 to define 32 or 64 bit signed integer.For example, 56 and -29.
- Bool − This represents a Boolean value which is either true or false.
- String − This is an ordered collection of characters. For example, "BUIE Learning"
- Double − This is used to represent a 64-bit floating-point number and used when floating-point values must be very large.
- Character − This is a single-character string literal. For example, "J"